Select Lesson
Overview
What are turtle graphics and other modules
5 mins
Reference list
5 mins
Sequences
5 mins
Coordinates
5 mins
Drawing graphics back to back activity
20 mins
Loops
5 mins
Lists
5 mins
Intro Turtle Graphics - Lesson 1. Turtle Challenge
15 mins
Lesson 5. Turtle graphics
30 mins
Lesson 6. Turtle drawing basics
15 mins
Moving
5 mins
Turning
5 mins
Drawing/Coloring
5 mins
Circles
5 mins
Movement—absolute and relative (optional)
5 mins
Turtle Graphics
Overview
What are turtle graphics and other modules
5 mins
Reference list
5 mins
Sequences
5 mins
Coordinates
5 mins
Drawing graphics back to back activity
20 mins
Loops
5 mins
Lists
5 mins
Intro Turtle Graphics - Lesson 1. Turtle Challenge
15 mins
Lesson 5. Turtle graphics
30 mins
Lesson 6. Turtle drawing basics
15 mins
Moving
5 mins
Turning
5 mins
Drawing/Coloring
5 mins
Circles
5 mins
Movement—absolute and relative (optional)
5 mins
60 mins
Turtle graphics in Python
Turtle graphics are a fun intro to Python. We import a robotic turtle and then use commands move the turtle. As the turtle moves, it leaves a trail behind it. We can make some pretty cool shapes using this.
Guide
Overview
This lesson plan covers all you need to know about drawing with Pythonic Turtles. Firstly, it takes you through concepts and activities then it lists the Turtle lessons found on Code Avengers. There is some more in depth information listed as extension concepts. This is an in-depth break down of the content to enhance learning and help teachers unfamiliar with these concepts to be able to teach them.
Key to lesson icons
There are 3 types of lesson segments
Concepts - are notes to expand on learning in lessons
Activities - are off-line and online games and activities to help your learning.
CA Lessons - are online lessons, projects and quizzes created by Code Avengers.
Extension: - These are additional segments for fast learners (or homework)
Concepts
What are turtle graphics and other modules
In the 1960s the code for Turtle graphics was created to help teach sequences and coordinates.
The code was originally in the Logo programming language developed by Wally Feurzig and Seymour Papert in 1966 and is now a common teaching tool in Python.
The turtles module can be easily
imported
from the Python library.
import turtle
variable = turtle.Turtle()
How to import the turtle
module
import turtle
tia = turtle.Turtle()
tia.forward(150)
tia.right(90)
The code above will:
- access the turtle module with
import
,
- create the turtle object and rename it Tia.
- then draw a line with
tia.forward(150)
,
- rotate Tia in place 90 degrees clockwise,
tia.right(90)
.
- rotate Tia in place 90 degrees clockwise,
- then draw a line with
- create the turtle object and rename it Tia.
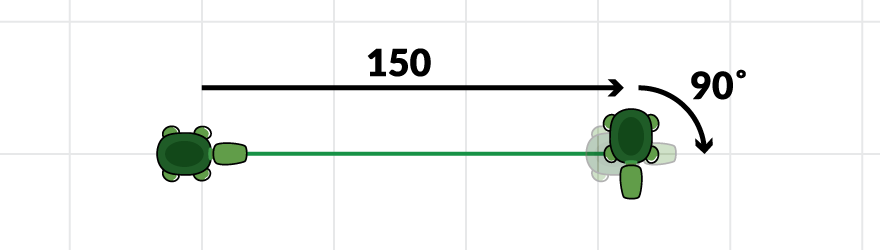
What is a module?
A module is a file containing Python code. Various modules do different things and they can be easily accessed by importing them into your program. Once imported you can call the functions in the modules and run them.
Modules store mini programs and information that you can access and use for your own programming.
Python has a library→ of standard modules.
Some cool beginner ones are:
random, which will generate random numbers
key word, this tests whether a string is a keyword in Python.
math has a lot of mathematical functions you can use (sin() etc.)
datetime will access basic date and time types.
These are some more modules →, for people with a bit more experience in Python.
Concepts
Reference list
Here is a quick list of the common turtle functions. You will find more information on each section below.
Move (relatively*)
.backward(pixels)
.forward(pixels)
.right(angle)
.left (angle)
Move (absolutely*)
.goto(x,y)
.setHeading(angle)
#0 = face right, 90 = face upwards, 180 = face left, 270 = face downwards
Drawing
.penup()
.pendown()
.pensize(pixels)
.pencolor("name") or (rgb(255,255,255) or (#FFFFFF)
.fillcolor("name") or (rgb(255,255,255) or (#FFFFFF)
.color("name") or (rgb(255,255,255) or (#FFFFFF)
.begin_fill()
.end_fill()
Circles
.circle(radius)
.circle(radius, angle)
#Circles always draw to the left of the way the turtle is facing.
Concepts
Sequences
A sequence is just a fancy name for a list that must be followed in order.
The computer needs to be told what to do in precise order.
Every step needs to be carefully written and in the proper order.
The most common example of sequence is writing a recipe.
In a recipe you complete each instruction before moving onto the next one and changing the order may change the results.
For example, you will have a very different cake if you added egg to the cake after baking it, instead of before baking it.
We can see the same thing in the code examples below.
tia.forward(10)
tia.right(90)
tia.forward(10)
tia.right(90)
tia.forward(10)
tia.right(90)
The code above will create a square.
The code below will create a long line.
tia.forward(10)
tia.right(90)
tia.right(90)
tia.forward(10)
tia.forward(10)
tia.right(90)
Break down the code
Take this forward function that moves the turtle and draws a line:
tia.forward(10)
tia.right(90)
- We need to say what object we want to perform the action, here it is
tia
, - What is the object doing (moving forward 1 pixel).
- We can repeat the order by adding a number in the brackets (10 pixels).
- Because the
right()
function is written beneathforward()
it will happen after the first bit of code has run. - This time the number in the brackets changes the angle of the turtle.
Note that each function() will need different information in the brackets: forward() needs pixels, right() needs an angle and goto() needs coordinates.
Concepts
Coordinates
When the code is run it appears in a little drawing box we call a canvas. That canvas is made up of 400 pixels by 400 pixels.
(That is our size of canvas, canvases can be any size you set them)
To place your turtle at a specific place on the screen you simply need to write the coordinates- we have a helpful grid on ours to help with placement.
Coordinates
Coordinates are pairs of numbers, like (3,4). They help you find places on a grid.
The first number tells you the horizontal position of a point, it tells to you go across the x axis until you hit the number 3.
The second number tells you the vertical position of a point, it tells to you go down the y axis until you hit the number 4.
Coordinates are used in both mathematics and computer science, (as well as in geography, archeology, criminology, the army, and many other areas). But they are used in different ways.
Math uses an axis with 0, 0 in the middle, and counts across and up. Up from 0 are positive numbers on the y axis and when you count down from 0 you have negative numbers. The same is true for left and right. Left of 0 are negative numbers and right of 0 are positive numbers. The coordinates (0,0) or (3,4) give the location of a point. (shown in the image as the points where 2 lines cross)
Computer grids model a computer screen, which is made up of thousands of little squares called pixels.
You can't have negative pixels, so 0,0 starts in the top left corner of your screen.
When given computer coordinates you count across the x axis from left to right, just like reading a book and then down the y axis. The coordinates (0,0) or (3,4) point to a specific pixel. (shown in the image above as a square)
Imagine the above example is very [[zoomed in- pixels are tiny]]
The CA course Food Frenzy→ uses a grid to place game objects. You can change the numbers in the coordinates to change the location of the object.
Activity
Drawing graphics back to back activity
This is a drawing activity on paper that is done in pairs. Learn about coordinates, grids, and being how those techniques help you to be precise. This activity is suitable for 8 year olds and up.
Objective
This pair-activity helps students to learn to give and follow precise instructions in order to draw simple graphics and demonstrate the precision of coordinates.
Background
All complex computer graphics start with instructing the computer to draw basic shapes at precise locations.
Coordinates
Learners should use coordinates to help them explain how to draw the pictures.
Coordinates are pairs of numbers, like (3,4). They help you find places on a grid.
The first number tells you the horizontal position of a point, it tells to you go across the x axis until you hit the number 3.
The second number tells you the vertical position of a point, it tells to you go down the y axis until you hit the number 4.
Below you can see the position of the coordinates (3,4).
Preparation
- Print off enough worksheets for each pair to have a copy.
- You can print 2 A4 pages or, to save paper, you can print of 1 A4 page that you will need to cut in half.
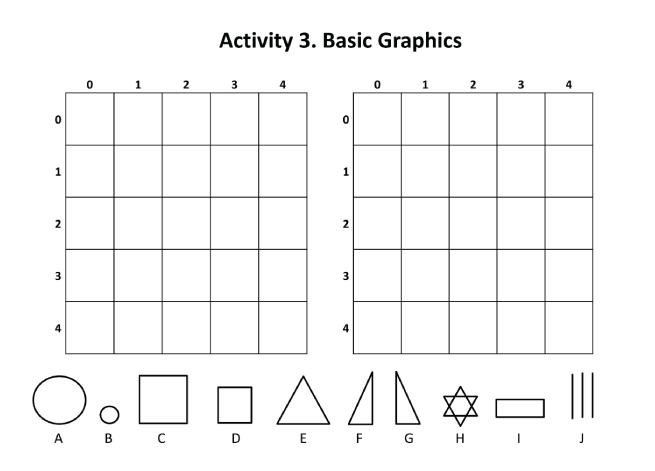
Instructions
Intro: Freehand Drawing (optional, extra 5 min)
All learners, either on a blank piece of paper (with no grids) or online on this blank drawing canvas at /javascript/94, will try to draw a simple picture that the instructor will make up and call out.
Example dialog:
- In the top left of the page draw a circle,
- In the middle of your page draw two squares stacked on top of each other.
- Add a bigger triangle on top.
- Add a small circle inside the top square, in the top right corner.
- Draw a long rectangle inside the bottom square, attached to the bottom.
- Add two lines to the bottom of the bottom square, going off the page.
The instructions create a very simple house
Get learners to compare their results- are they identical?
Is everything the same size and in the same place?
If not discuss why not.
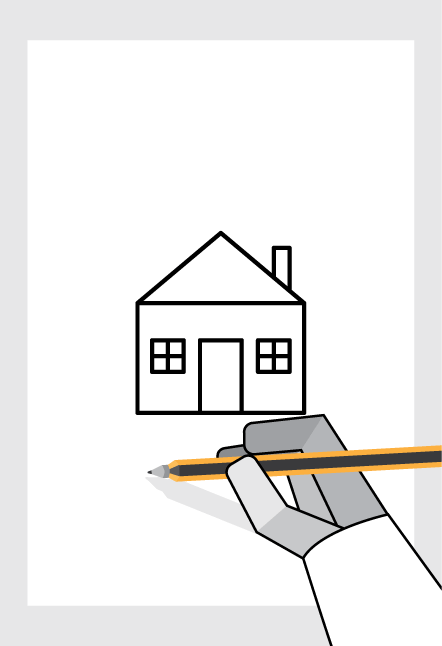
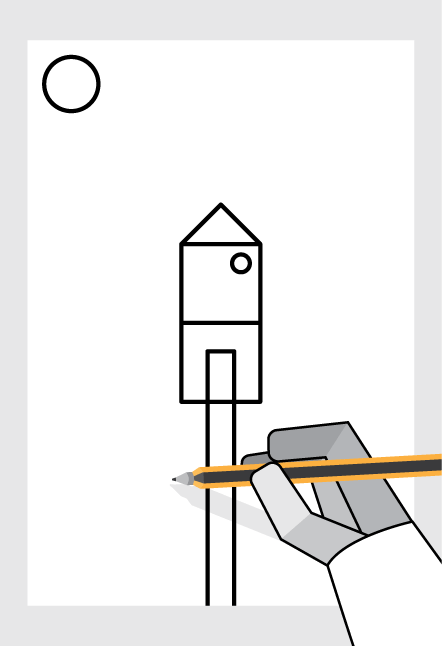
Activity:
- Players will be in pairs, sitting back to back.
- Decide who is Player 1 and who is Player 2.
- Explain that the players are drawing images from instructions and these images have to be accurate.
There will be two rounds.
Round 1:
Player 1 will call instructions and Player 2 will draw.
Round 2
Then, Player 2 will call instructions and Player 1 will draw.
- Give Player 1 the Player 1 page and Player 2 the Player 2 page. They must not see each others page.
- To start, those with "Instruct 1" in the first grid are Player 1 and they will give clear instructions to Player 2 to draw image one.
For example;- "Player 2, in the DRAW 1 grid, draw shape A in the square (4, 0)."
- "Draw shape F in the bottom right corner of (1, 3)."
- Once the pair completes the 1st shape, they can swap roles. (Don't look yet!)
- Player 2 describing "Instruct 2" and Player 1 drawing in "Draw 2".
- Compare drawings.
- Ask questions about what happened and what worked/didn't work.
Was it more accurate than the house drawing in the intro?
Versions:
- Easy: Player 1 can tell Player 2 what the picture is of. You could also print a few copies of the bottom row of shapes and students can paste them rather than draw them.
- Medium: Students can only give instructions, not say what the image is.
- Hard: Cut off the shape directory at the bottom of the page and players must describe the shapes along with their sizes and orientations.
Answers
Find the answers here
Concepts
Loops
There are 3 basic ways that computers read code.
The first is in sequence, where the code is read line after line in order (you can read more on that above).
The second is called conditional, where code could be run or ignored depending on true/false statements.
And the third is sometimes called iterative, which is a fancy word for repeating code.
Loop functions fall into the third category. They are really fun because they allow you to draw complicated images with only a few lines of code.
There are two kinds of loops, for loops
and while loops
.
Turtle graphics can use for loops
to make interesting patterns or automatic color changes..
For loops look like this:
for i in range(4):
print('Hello')
This loop would repeat 4 times because of the range(4)
part.
To use loops with turtle graphics, you have to think carefully about which steps need to be repeated.
These steps go inside the loop where it says print('Hello')
and they are indented to show they are inside the loop.
To draw a regular shape, inside the loop you will need the line of code that moves your turtle forward, and the line that turns her the correct angle.
Drawing shapes with loops
The big part of drawing shapes with loops is to work work out what is the repeating angle.
To work out angles on
regular
shape is pretty easy, it only uses a little bit of math.
A whole complete shape has 360 degrees, that means that the angles inside the shape have to equal 360 degrees.
So simply count the corners of your shape and divide 360 by that number:
Shape | Corners (n) | Equation | Degrees (d) | for i in range(n):
|
Triangle | 3 | 360/3 = | 120° | for i in range(3):
|
Rectangle | 4 | 360/4 = | 90° | for i in range(4):
|
Pentagon | 5 | 360/5 = | 72° | for i in range(5):
|
Hexagon | 6 | 360/3 = | 60° | for i in range(6):
|
Dodecahedron | 12 | 360/12 = | 30° | for i in range(12):
|
for car in range(4):
Is an odd but perfectly fine thing to write.Concepts
Lists
We can use a number to run a loop, as we have seen when using for i in range(4):
. Another way we can run a for loop is using a list.
A list is a set of values stored together.
The list, square brackets with commas, just replaces the range().
for i in [0, 1, 2, 3]:
tia.forward(50)
tia.right(90)
Will also run the code 4 times because there are 4 numbers inside the list.
But doing the same thing is not interesting.
What is really cool is that you can put different numbers inside the list and each one will be run once.
Lets make a crazy line by changing the angles of our code each time the loop is run.
We have to simply add the list of angles and put the variable i inside the function where the angles are normally written.
for i in [30, 40, 50, 60]:
tia.forward(50)
tia.right(i)
tia.forward(20)
tia.left(90)
Set the list in a variable
Variables are useful to use for lists because they make the code easier to read and it is easier to make changes if you use the list multiple times.
lengths = [10, 20, 30, 40, 50, 60, 70, 80, 90, 100, 110, 120, 130]
for length in lengths:
tia.forward(length)
tia.right(90)
In this example we have a list of lengths. To create the loop we write: for length in lengths:
This lets us use each value in the list by typing the word length wherever we want to use it. The loop repeats once for each value making this cool shape:
Change pensizes
You have to use penup()
and pendown()
to reset the pensize
pensizes = [5, 10, 15, 20, 25, 30, 25, 20, 15, 10, 5]
for pen in pensizes:
tia.penup()
tia.pensize(pen)
tia.pendown()
tia.forward(30)
Change colors
# Create a list of colors
colors = ["red", "blue", "gold", "green"]
# Use a loop to draw a square with one side each color
for color in colors:
tia.color(color)
tia.forward(320)
tia.right(90)
Combine pensizes and color'
# Create a list of colors
colors = ["red", "blue", "gold", "green"]
pensizes= [5, 10, 15, 10, 5]
# Use a loop to draw a square with one side each color
for i in range(4):
for pensize in pensizes:
tia.penup()
tia.pensize(pensize)
tia.pendown()
for color in colors:
tia.color(color)
tia.forward(17)
tia.right(90)
Python 1 Project
Intro Turtle Graphics - Lesson 1. Turtle Challenge
Lesson Outline
task 0: Turtle Challenge
task 1: Introduction to Python Turtles
task 2: Changing the style of the lines
task 3: Moving to an exact position
task 4: Filling shapes with colors
task 5: Draw the flag of Mali
task 6: Using loops to repeat code
task 7: Draw a Rubik's cube
task 8: Using a list to run a loop
task 9: Drawing circles
task 10: Drawing semicircles
This is our easy simple beginners project that lets you have fun and get quickly into Pythonic Turtles.
Python Intro Lesson
Lesson 5. Turtle graphics
Lesson Outline
task 0: Turtle graphics
task 1: Draw with Tia the Turtle
task 2: Move and turn Tia
task 3: Change the color and size
task 4: Draw some different shapes
task 5: Filling shapes with colors
This is a short intro lesson on Turtles that is part of a wider introduction to Python
Python 1 Lesson
Lesson 6. Turtle drawing basics
Lesson Outline
task 1: Meet Tia the Turtle
task 2: Move and turn Tia
task 3: Change the color and size
task 4: Draw some different shapes
task 5: Filling shapes with colors
Our Python one course teachers the important first steps of coding and breaks it up with this enjoyable graphic module.
Extension: Concepts
Moving
Moving your turtle is easy. You can either tell your turtle to go forwards or backwards from its current position or tell it to go to a different place on the canvas.
Forward and backwards (relative*)
We can tell the turtle to move forward or backwards one pixel from it's current location with
turtle.forward()
and turtle.backwards()
Note: it will move forwards in the direction it is facing.
Now one pixel is not a useful distance to travel to draw graphics.
To make our turtle move further add a number into the brackets turtle.forward(100)
Move to a coordinate (absolute*)
The turtle is sitting inside a canvas with a grid. Using that grid we can give coordinates for the turtle to move to.
turtle.goto(100,50)
will move the turtle across to column 100 and down to row 50 on the grid. (Where the turtle was before doesn't matter)
Extension: Concepts
Turning
Straight lines can only take you so far and turning is just as simple.
Turn to the right or the left (relative*)
you can use turtle.left()
and turtle.right()
to face your turtle in a new direction.
It uses [[angles]- any number between 0 and 360.
Give the command turtle.left(25)
, and the turtle rotates in place 25 degrees clockwise. Generally we use 90° to turn.
Face a direction (absolute*)
SET HEADING
turtle.setheading()
uses angles to face the turtle to a set direction.
It doesn't matter what direction our turtle is facing to begin with, turtle.setheading(90)
will face him up to the top of your screen and turtle.setheading(180)
will face your turtle ot the left of the screen.
setheading
we go anticlockwise and use what is called Standard Mode where 0 is the right side of the screen (East) →.
Anticlockwise |
0 - Right side of screen → |
90 - Top of screen ↑ |
180 - Left side of screen ← |
270 - Bottom of screen ↓ |
Extension: Concepts
Drawing/Coloring
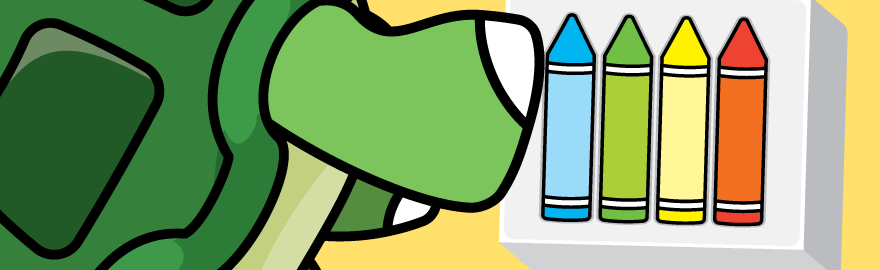
Pen
The robotic turtle is dragging a pen that can change color and size.
You can change the width of the pen to any positive number (though 20+ might be getting a bit big to draw with).
The code looks like:
turtle.pensize(5)
turtle.pensize('auto')
will set the pensize to 0.
There are times when you you want to move the turtle without leaving a line.
We use turtle.penup()
to stop drawing so you can move your turtle or change the pensize and turtle.pendown()
to start drawing again
Head ups!
If you want a line of different pensizes you have to put in pauses, using penup() and pendown()
, to do this.
import turtle
tia = turtle.Turtle()
tia.penup()
tia.goto(100,100)
tia.pendown()
tia.pensize(5)
tia.forward(50)
tia.penup()
tia.pendown()
tia.pensize(10)
tia.forward(50)
tia.penup()
tia.pendown()
tia.pensize(15)
tia.forward(50)
tia.penup()
tia.pendown()
tia.pensize(20)
tia.forward(50)
Stroke and fill
Changing colors can make your images more interesting and complex.
There are two areas in a shape that we can color
fill
'Color in', the color that fills up the inside area of a letter or shape
stroke
The lines that make up the outline of a letter or shape
This square has a red stroke and a yellow fill.
You can set the pen color, the fill color or simply the color, which will set both the stroke and the fill. The fill only works when you tell the code to begin filling and you have a whole shape to color in. (it wont work on a line).
Stroke color —.pencolor()
import turtle
tia = turtle.Turtle()
tia.pencolor('red')
tia.fillcolor('yellow')
tia.begin_fill()
for i in range(4):
tia.forward(50)
tia.right(90)
tia.end_fill()
Fill color —.fillcolor()
#fill is set
tia.color('blue')
for i in range(4):
tia.forward(50)
tia.right(90)
tia.forward(80)
#fill starts
tia.begin_fill()
for i in range(4):
tia.forward(50)
tia.right(90)
tia.end_fill()
Colors
There are a couple of ways you can change the color of your graphic.
You can use 'symbolic names' like 'red', 'chartreuse' 'OrangeRed', or, if you know about RGB or Hex colors, these work too.
turtle.color('steelBlue')
Nameturtle.color(rgb (88, 137, 210))
) RGBturtle.color('#5889D2')
Hex
Here are some color names:
Extension: Concepts
Circles
Python turtles also have a built in function called circle()
which, you guessed it, let's you draw circles.
The code that follows will draw a circle with a radius (half the circle's width) of 60px: tia.circle(60)
.
The circle is always drawn on the turtle's left. If the turtle is facing a different direction, the circle will be drawn in a different place.
To fill a circle, do the following: tia.begin_fill() tia.circle(50) tia.end_fill()
Semi circles
We can also draw semicircles by putting a second number inside the brackets: tina.circle(60, 180)
This code draws half of a circle, because we've told the turtle to only draw 180 degrees of it.
Because circles are always drawn on the turtle's left, sometimes we might want to tell the turtle exactly which direction to face, using setheading(90)
is very helpful here to point the turtle straight up.
Extension: Concepts
Movement—absolute and relative (optional)
Absolute and relative are two different ways to talk about directions.
Absolute | Relative |
Absolute directions use fixed positions- the top, bottom, left side and right side of the screen (or North, South, East and West, meters above or below sea level). | Relative directions are based on the position of the object or person. There is forward, backwards, up, down, left and right. |
We can illustrate this more by explaining that there are often two different ways of giving directions. | |
![]() | ![]() |
Directions:
1. "Face north on First St, drive to Second St, 2. Turn to face west, 3. Drive to Food Avengers, 4. Face north to enter." | Directions:
1. "Go forward up this road we are on for 10 min, 2. Turn right and 3. Go down that road for 5 min, 4. Food Avengers is on the left." |
The first set of directions are how you read a map and the second might be used by a helpful stranger or your GPS. Both work as directions.
What is the difference? | |
The first example shows something called absolute. The directions on it have nothing to do with you, instead they are connected to the area around you. As long as you are on Earth, North is always a set direction. If you are backwards, frontwards or upside down, it does not change the direction of North.
The corner of 1st street and 2nd street is exact point, it doesn't rely on how far you have traveled or what direction you are facing to know where it is. | The second example is relative to you. The instructions centered around you (or the object moving). It doesn't matter what way you are facing, "right" is always on your right side and forward is always the way you are facing. |
In coding, it is useful to know the difference between code that is relative to the object or absolute.
Python | JavaScript | |
Absolute | talia.goto(300,200)
| new Game.GoodItem(30, 220)
|
Relative | talia.forward(30)
| robot.forward(3)
|
Resources
Guide
- download
- new file
- upload media
- rename
- delete
Run Ctrl+Enter
Check Ctrl+Shift+Enter
Reset Ctrl+Backspace
Redo Ctrl+Y
Cut Ctrl+X
Copy Ctrl+C
Paste Ctrl+V
Find Ctrl+F
Find & replace Ctrl+F+F